Maybe you are a beginner in coding and you have heard about functions and you quickly want to know what it means, you have come to the page that will holistically look at Functions in programming and how to use them.
As a coder or a programmer, you need to know what “function” means as it makes your coding experience more efficient and easier. I used to be confused when I hear the word function during my early days in tech. So, if you were like me, not to worry, you will complete this blog post happy and equipped.
This article is great for anyone who is inexperienced or has zero knowledge about what function is in programming. Also, it can widen the knowledge of an intermediate in programming and help the advanced to be up to date with changes and methods.
Let’s start with the basics.
What is a Function in Programming?
In computer programming, a function is a standalone section of code that completes a single purpose and can be accessed by other program elements.
Code can be made more modular and simpler to comprehend, develop, and maintain by using functions. They can be used repeatedly within a program and have the ability to receive input and return output values.
In general, functions are made to make coding easier and to better organize and structure it.
Functions in programming: Importance
- Programming introductory courses frequently refer to functions as “black boxes.” In other words, when a programmer uses a function, they are just interested in the outcome and don’t care what the code within it actually performs.
- Functions make it possible for the reuse of codes in your program. As long as you have been able to give the function a definition, it can run the exact way in any part of your program which saves time and makes the program simplified.
- With Functions, a programmer can debug programs more easily because everything has been logically broken down into functions. They can narrow down the problem to a single function rather than having to sift through the entire program to find it.
What are the types of functions in programming
In programming, there are various types of functions that you can use to organize and structure your code. Here are a few commonly used types:
- Built-in Functions: These are functions that come pre-defined in programming languages and can be directly used without needing to write their implementation. Examples include print() for outputting text, len() for finding the length of a string or list, and input() for accepting user input.
- User-defined Functions: These are functions that you create yourself to perform specific tasks. You can define their behavior, input parameters, and return values. User-defined functions are great for breaking down complex problems into smaller, manageable parts, and improving code reusability. You can name your functions anything you like!
- Recursive Functions: These are functions that call themselves, allowing a function to repeat its own execution until a certain condition is met. Recursion is often used to solve problems that can be divided into smaller, similar subproblems. For example, calculating the factorial of a number using recursion.
- Higher-order Functions: These are functions that can take other functions as arguments and/or return functions as results. They are widely used in functional programming paradigms and allow for powerful abstractions and code flexibility.
- Lambda functions: Also known as anonymous functions are a concise way to define small, one-line functions without explicitly giving them a name. They are commonly used in situations where a function is needed as an argument to another function or for simple, short-lived operations.
Here’s an example to illustrate how a lambda function can be used with map() to square each element in a list:
numbers = [1, 2, 3, 4, 5]
squared_numbers = list(map(lambda x: x**2, numbers))
print(squared_numbers) # Output: [1, 4, 9, 16, 25]
Parts of functions in programming
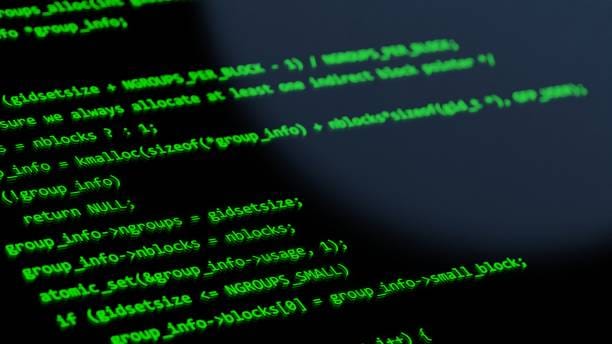
Functions are composed of several elements that define their behavior and how they interact with the rest of the program. Here are the specific components that make up a function:
- Name: A function has a name that uniquely identifies it in the program. This name is used to call or reference the function when needed.
- Parameters: Functions can have zero or more parameters, which are placeholders for values that can be passed into the function when it is called. Parameters allow functions to receive input values and work with them.
- Return Type: A function can have a return type, which specifies the type of value that the function will produce as output when it is called. Not all functions have a return type; some functions may perform actions or modify data without returning a value.
- Body: The body of a function contains the statements or codes that define what the function does. It represents the instructions or operations that will be executed when the function is called. The body can include variable declarations, control structures (like loops or conditionals), and other function calls.
- Input Arguments: When a function is called, input values called arguments can be passed into the function’s parameters. Arguments provide the actual values that will be used by the function during its execution.
How to write functions in programming
- Function Declaration/Definition: Start by declaring or defining the function using the syntax specific to the programming language you are using. This includes specifying the function name, parameters, and return type (if applicable). For example, in the Python programming language, you are using. This includes specifying the function name, parameters, applicable). For example, in Python:
def greet(name):
# Function body goes here
print(“Hello, ” + name + “!”)
- Function Body: Within the function, write the code that defines the desired behavior. This code will be executed whenever the function is called. You can include any statements, logic, or computations necessary to perform the intended task. For example:
- Function call: to actually use the or invoke the function, you need to call it by its name, providing the appropriate arguments (if any). The function will then execute its code and produce a result( if applicable). For example
Functions in Java
Functions are known as methods and they reside within classes. Here are the steps to write functions in Java:
- Method Signature: Start by declaring the method using the following syntax: java
( ) { // Method body goes here } - <access_modifier>: This specifies the visibility of the method, such as public or private. It determines which parts of the program can access the method.
- <return_type>: This indicates the type of value that the method will return after execution. If the method does not return a value, use the keyword void.
- <method_name>: This is the unique identifier for the method.
- <parameter_list>: This is a comma-separated list of input parameters, each with its own data type and name.
- Method Body: Within the method, you write the code that defines the behavior of the method. This code will be executed whenever the method is called. You can include any statements, logic, or computations necessary to perform the intended task.
- Return Statement: If the method has a return type
Functions in Python
- Function Definition: Start by using the def keyword, followed by the function name and parentheses (). If the function takes any parameters, specify them within the parentheses. Finally, end the line with a colon: to indicate the start of the function’s body. Here’s an example:
def greet(name):
# Function body goes here
print(“Hello, ” + name + “!”)
- Function Body: Within the function, write the code that defines the behavior of the function. This code will be executed whenever the function is called. Use indentation (typically four spaces or a tab) to denote the block of code that belongs to the function body. For example:
def greet(name):
print(“Hello, ” + name + “!”)
print(“Welcome to our website!”)
- Function Call: To actually use or invoke the function, you need to call it by its name, followed by parentheses (). If the function takes any arguments, provide them within the parentheses. For example:
greet(“John”)
This will execute the code inside the greet function and print “Hello
Functions in C++
To write functions in C++, follow these steps:
- Function Declaration: Declare the function prototype before using it in your program. The function prototype consists of the function’s return type, name, and parameters (if any). For example:
int sum(int a, int b);
- Function Definition: Define the function body, which contains the actual implementation of the function. It should be written after the declaration and before the main function or any function that calls it. For example:
int sum(int a, int b) { int result = a + b; return result; }
- Function Call: In your program, call the function whenever you need to use it. Pass the required arguments (if any) inside the parentheses. For example:
int x = 5, y = 3; int addition = sum(x, y);
check out this complete example:
In this example, the sum() function takes two integer arguments (a and b), adds them together, and returns the sum. The main() function calls sum() with the values of x and y, and then prints the result.
Remember to include any necessary headers at the beginning of your program, such as <iostream> for input/output operations in this example.
What is an Objective Function in Linear Programming?
In linear programming (LP), an objective function is a mathematical expression that needs to be maximized or minimized. It represents the goal or objective of the optimization problem. Linear programming is a mathematical technique used to find the best outcome in a mathematical model that is subject to certain constraints, which are represented as linear relationships.
The general form of a linear programming problem can be defined as follows:
Maximize (or Minimize) Z = c1x1 + c2x2 + … + cnxn
subject to:
a11x1 + a12x2 + … + a1nxn ≤ b1
a21x1 + a22x2 + … + a2nxn ≤ b2
…
am1x1 + am2x2 + … + amnxn ≤ bm
x1, x2, …, xn ≥ 0
In this formulation:
- Z is the objective function, and it represents the quantity that needs to be maximized or minimized. The coefficients c1, c2, …, cn are constants representing the contribution of each variable x1, x2, …, xn to the objective function.
- x1, x2, …, xn are decision variables that represent the quantities to be determined in the optimization process. These are the values that we are trying to find to optimize the objective function.
- a11, a12, …, amnxn are coefficients representing the constraints that the decision variables must satisfy.
- b1, b2, …, bm are constants representing the right-hand side of the constraints, and they determine the limitations or restrictions imposed on the decision variables.
The goal of the linear programming problem is to find the values of x1, x2, …, xn that maximize (or minimize) the objective function Z while satisfying all the given constraints.
The constraints ensure that the solution remains feasible within the specified bounds. Linear programming is widely used in various fields, including economics, operations research, logistics, and resource allocation problems.
Conclusion
You should be aware that whenever a function is called in programming, the program stops for the duration of the function’s execution. The software is therefore kept in memory that is currently being used.
You use more active memory if you call more functions without finishing them. Your software might spiral out of control if you are not careful.
Frequently Asked Questions
A function in programming is a reusable block of code that performs a specific task or set of tasks. It allows you to encapsulate functionality and give it a name, making it easier to organize and maintain code. Functions can take input parameters, perform operations based on those inputs, and return a result as output.
In most programming languages, a function is defined using the following syntax:
def function_name(parameter1, parameter2, …):
# Function body – code that defines what the function does
# It may involve operations on the parameters or other logic
return result # (optional) Return statement to provide output
Using functions offers several benefits, including:
Reusability: Functions can be called multiple times from different parts of the code, reducing redundancy and promoting modular design.
Readability: Functions help make code more readable by breaking complex tasks into smaller, manageable units.
Abstraction: Functions hide implementation details, allowing users to focus on what the function does, not how it does it.
Testing and Debugging: Functions simplify testing and debugging as issues can be isolated to specific functions.
Scoping: Functions create their own scope, preventing variable name clashes between different parts of the program.
Yes, functions in many programming languages can return multiple values. In some languages like Python, you can use tuples to return multiple values from a function:
def get_coordinates():
x = 10
y = 20
return x, y
Calling the function and unpacking the returned values
x_coordinate, y_coordinate = get_coordinates()
print(x_coordinate, y_coordinate) # Output: 10 20
Recommendation
- Top 12 Best Coding or Programming Schools In Lagos
- T0P 10 Best CNC Programming Courses to Learn in 2024
- Top 10 Best CNC Programming Schools In 2024
- CNC Programming: What it is and Why You Should Learn it in 2024
- Programming Fundamentals For Kids: An Introduction
- 8 Best Coding Games for Kids that Teach Real Programming Concept